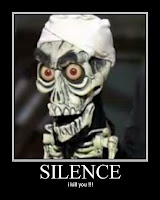
It’s going to be an ASP.net MVC4 website.
The content is secret;)
So today I was looking for the right DB for the site. Oracle and SQL Server were instinct choices. However as many of you I’m also subscribed to Ayende @ Rahien blog. And so as you I receive at least one raven db post a day. After reading a while I realized that RavenDb gives me the same abilities as a standard rational database and more (there too many articles about the efficiency of NoSql so I don’t want to add another one to the list).
That I do want is to guide you step by step in setting the RavenDb server and using the RavenDb Client in your application.
So let’s go!
RavenDb has a client and a server side. The client enables you to connect to the RavenDb server. The server can run in several ways:
Win Service
IIS Service
Embeded in the application.
The embedded option is for really small projects or for demos as is in real application you would want to run the RavenDb on a different server and configure it to suite your needs.
I want to make this tutorial for real application’s scenario so we’d choose the Win Service option.
Download the last stable build of RavenDb. In the zip file you’ll find some samples and a server folder, copy it to a suitable location for a database (c:/temp).
In the server folder there’re two files that you are interested in: Raven.Server.exe and Raven.Server.exe.config. In the config file you can specify a custom port for the server to run and the location of the Data Directory where Raven writes the data. Yes, RavenDb is an in-memory database however it writes the data to a file for backup and restore purposes.
<add key="Raven/Port" value="*"/>
<add key="Raven/DataDir" value="~\Data"/>
By default the port will be 8080 and the data directory will be stored inside the server directory (perhaps c:/temp wasn’t such a good idea).
Open the cmd and run the “…\Raven.Server.exe /install”.
Check you services.msc and you will find RavenDb service running.
A really cool feature is the management studio which is located on localhost:8080 (or your custom port).
Here you can see all your documents, indexes and logs. The logs tab allows you to see all the queries and the commands that Raven executes.
To enable it you need to create NLog.config file in the server directory and paste the following code:
<nlog xmlns="http://www.nlog-project.org/schemas/NLog.netfx35.xsd" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"> <targets> <target xsi:type="HttpEndpoint" Name="HttpEndpoint" /> </targets> <rules> <logger name="Raven.*" writeTo="HttpEndpoint"/> </rules>
</nlog>Restart the process and now you have logs!
Getting the client
After we have the RavenDb server running it’s time to add the client and start playing with Raven from the application.
For this tutorial we’ll need an Asp.Net MVC 4 project (if you don’t know how to open one read this).
In the package manager console type “Install-Package RavenDB.Client -Version 1.0.971”, the reason I do it from the console and not from the package manager is because the latest RavenDb.Client package has a Newtonsoft.Json.dll with a lower version when the version of the Newtonsoft.Json.dll that comes with the MVC project. By specifying this RavenDb.Client version we receive ravenDb.Client dlls compiled with the newest Newtonsoft.Json.dll.
Configurating Raven from the application
Similar to NHibernate, RavenDb has a DocumentStore (sort of SessionFactory) which only needs to receive the connection string name. . Paste this code inside the Application_Start in the Global.asax:
Store = new DocumentStore { ConnectionStringName = "RavenDB" };
Store.Initialize();IndexCreation.CreateIndexes(Assembly.GetCallingAssembly(), Store);The third row adds custom created indexes from the assembly to the RavenDb Server (we’ll talk about it later).
Same as the Nhiberbate’s SessionFactory, the DocumentStore has to be singleton, making it static should work for now.
public static DocumentStore Store;
Add the connection string to the “connectionStrins tag in the web.config.
Almost every controller will need an access to the RavenDb Session so lets create a RavenBaseController which will be responsible for opening and closing the session.<add name="RavenDB" connectionString="Url=http://localhost:8080" />Session managment
1: public class RavenBaseController : Controller2: {
3: public IDocumentSession RavenSession { get; protected set; }
4:
5: protected override void OnActionExecuting(ActionExecutingContext filterContext)
6: {
7: RavenSession = MvcApplication.Store.OpenSession();
8: }
9:
10: protected override void OnActionExecuted(ActionExecutedContext filterContext)
11: {
12: if (filterContext.IsChildAction)
13: return;
14:
15: using (RavenSession)
16: {
17: if (filterContext.Exception != null)
18: return;
19:
20: if (RavenSession != null)
21: RavenSession.SaveChanges();
22: }
23: }
Basic Session Usages
var student = new Student {Name = "San Yang"};
RavenSession.Store(student);
You can check how is the class converted to a Json document using the raven
studio.
Similar to NHibernate (Store = SaveOrUpdate) and if the class has an id property Raven will generate a unique id (numeric or string). However if you store an object with nested objects in it, Raven won’t generate ids for them.
Queries are same as in NHibernate:
Knock yourself out.var school = RavenSession.Query<School>() .Where(x => x.Students.Any(y => y.Name == "jones")) .ToList(); var school = RavenSession.Query<School>() .Where(x => x.Name == "") .ToList();
Indexes
RavenDb’s philosophy is “we shall never make you wait” and so far they haven’t disappointed me. Perhaps the dynamic index generation helps them there.
RavenDb analysis every query that is executed on the session and creates a temporary index for it. If the query is executed several (configurable) times then the index becomes permanent.
So that should give you a nice place to start from.
More posts about advanced RavenDb usages and options are coming soon.